Karel Level 5
Description
OH NO! Karel's beepers have been scattered! They might look like this:
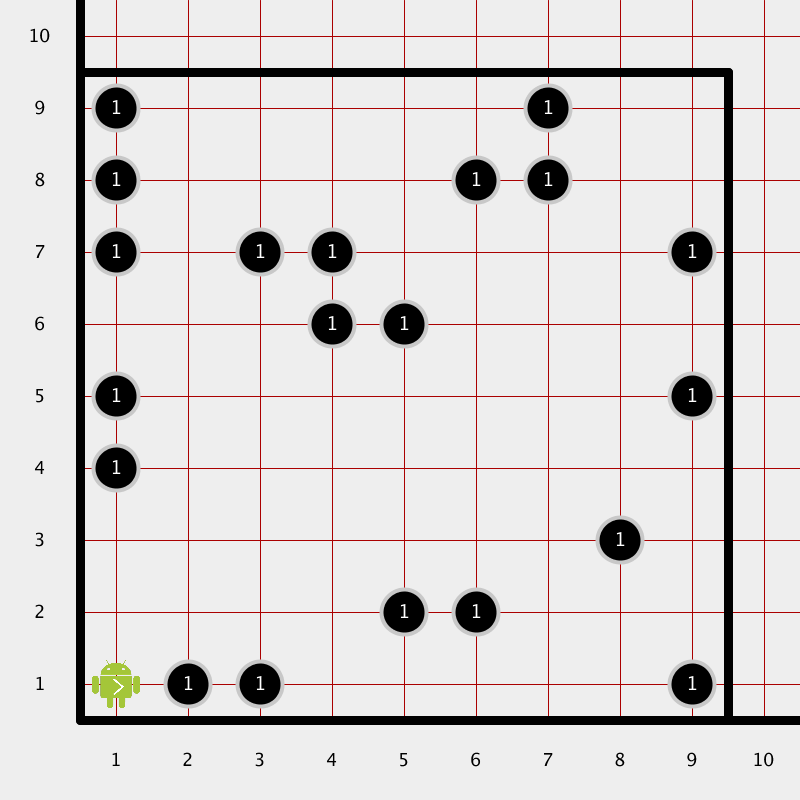
or like this:
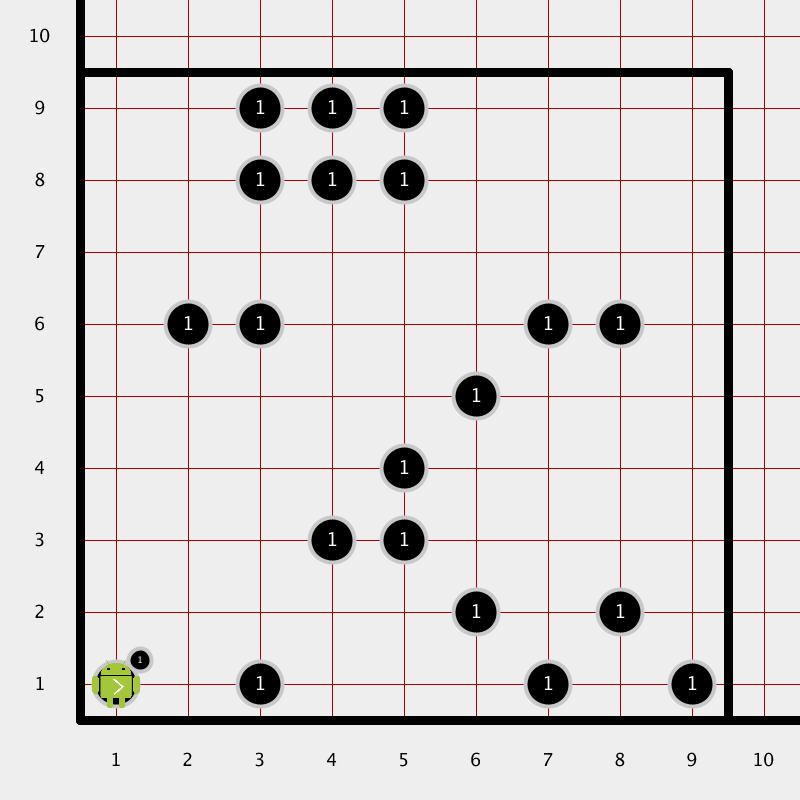
or any other random arrangement! Your job is to give commands to your robot so she will always clean up the scattered beepers, no matter where they actually are.
This problem can and should be solved with a Top-Down approach, if statements, and while loops ONLY. Do not use any other language features (for loops, variables, etc).
Solution
Part 1
This is what it should look like when you have successfully completed the first part of the level.
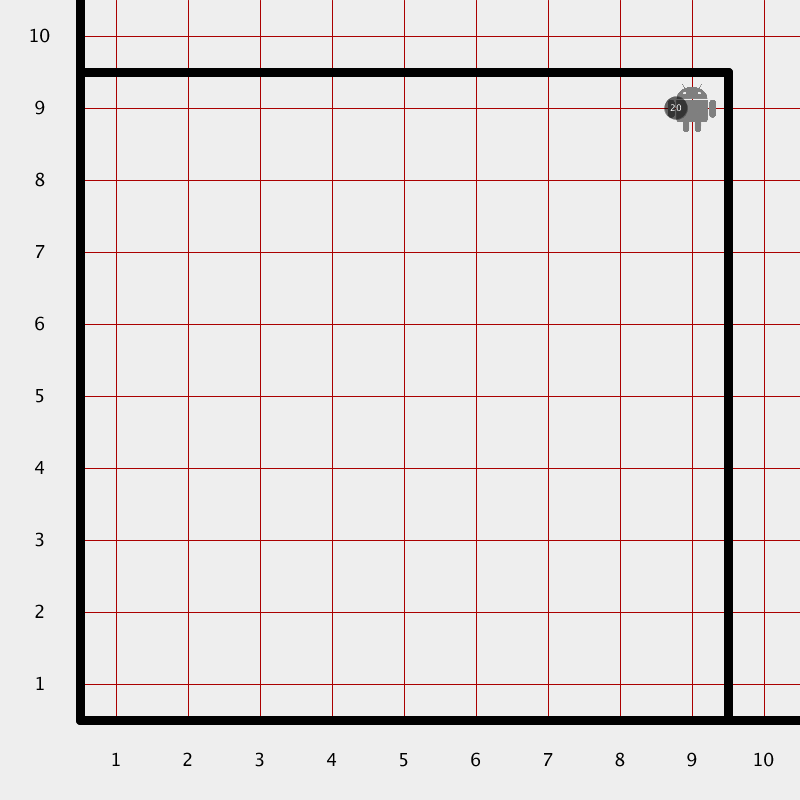
Part 2
Open the Level_5
tab and change line 4
from:
boolean loadMultipleRobots = false;
to:
boolean loadMultipleRobots = true;
That will load a level that looks similar to this:
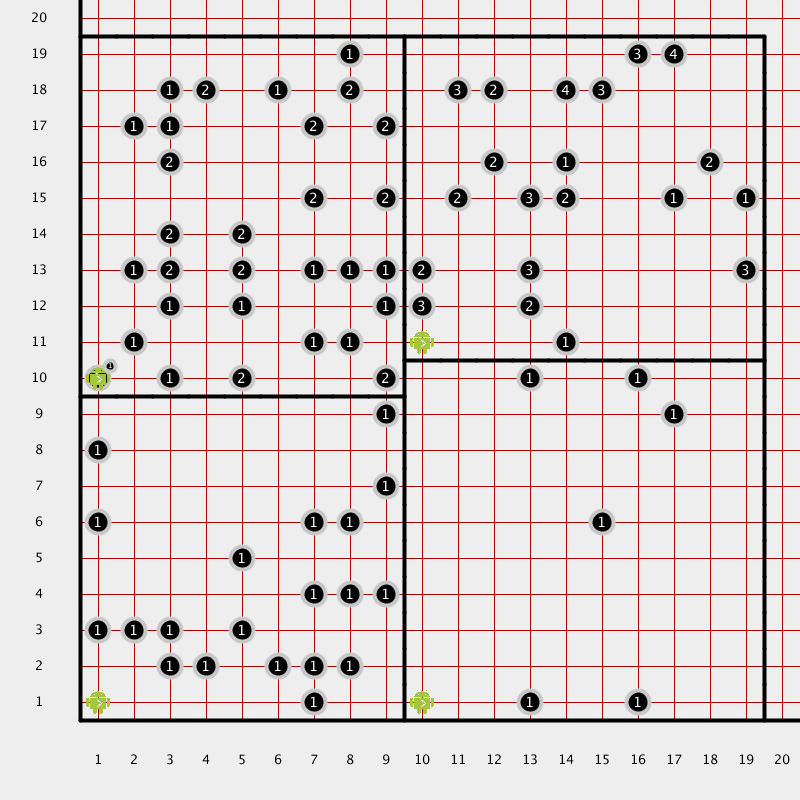
Each of the 4 robots must use the same set of commands to clean up their section of beepers. You are done when it looks something like this(the exact location of the robots and number of beepers each is holding may be different):
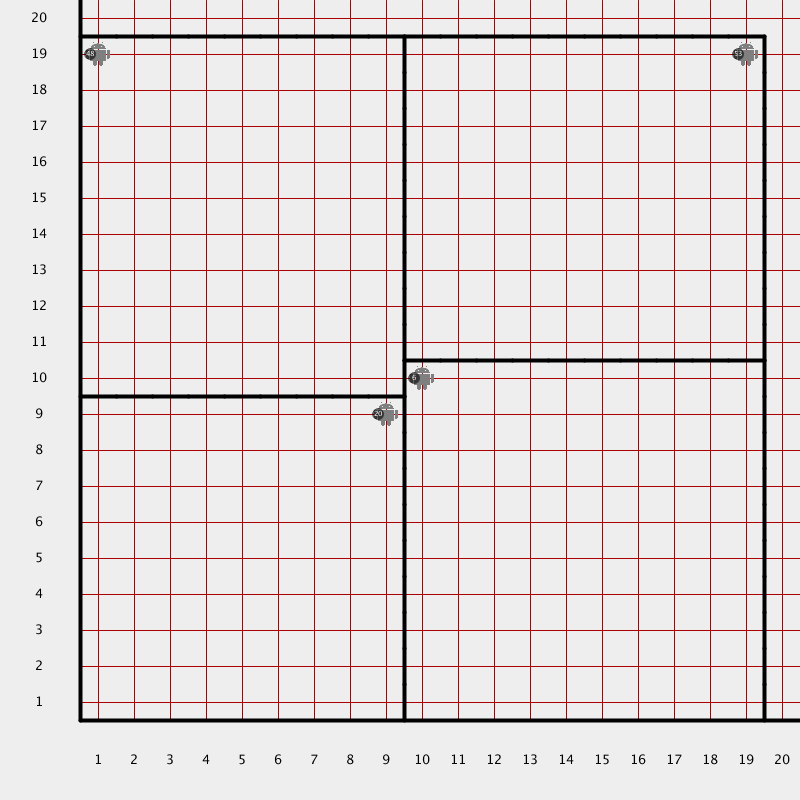
Results
The exact number of turns is not as important this time, but the moves should match exactly. This is only counted for the robot in the 9x9 grid.
Moves | 80 |
Picks | 20 |
Combining Ifs and Whiles
You may nest logic structures like If Statements and While Loops to solve more difficult problems. Examples:
// faceEast only when on a beeper
if (nextToABeeper()) {
while(facingEast()) {
turnLeft();
}
}
// face East, picking a beeper every
// turn if you can
while (!facingEast()) {
turnLeft();
if (nextToABeeper()) {
pickBeeper();
}
}
// pick a beeper if next to one and
// facing South
if (nextToABeeper()) {
if (facingSouth()) {
pickBeeper();
}
}
// keep moving to a wall until there
// is not a beeper next to that wall
while(nextToABeeper()) {
while(frontIsClear()) {
move();
}
turnLeft();
}
Use proper indentation to make it easy to see which blocks of code are associated with specific if and whiles.
Conditions
To help you solve this problem, you can ask Karel yes/no questions about the state of the world. Here are your choices:
- facingEast()
- Is the robot currently pointed to the East?
- facingSouth()
- Is the robot currently pointed to the South?
- facingWest()
- Is the robot currently pointed to the West?
- facingNorth()
- Is the robot currently pointed to the North?
- frontIsClear()
- Is there a wall directly in front of the robot?
- nextToABeeper()
- Is there at least one beeper on the same intersection as the robot?
- anyBeepersInBeeperBag()
- Is the robot currently holding any beepers?