Karel Level 4
Description
Four robots have each been trapped in a box, with only one way out. Help them escape!
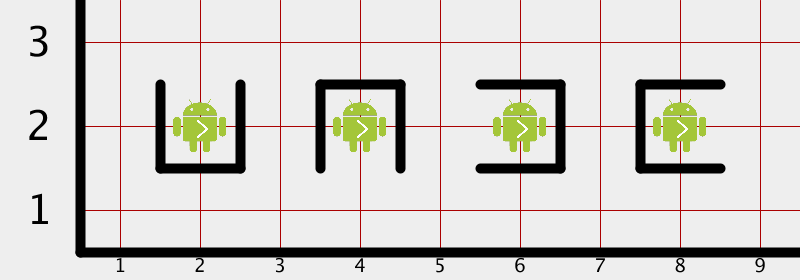
Solution
The robots should remove themselves from their boxes and end up facing East
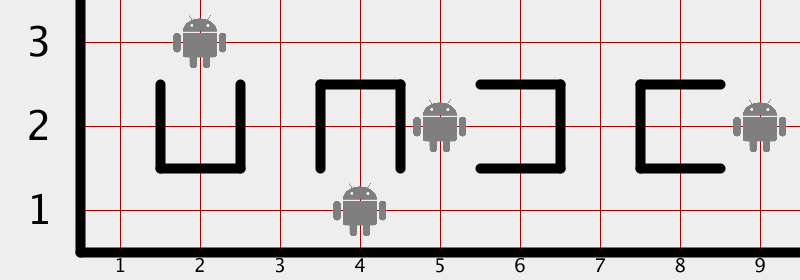
Your code should include a new faceEast() method that makes a robot face East no matter what direction it was facing previously.
Your solution may NOT include if statements!
While Loops
Sometimes you want to repeat some block code instead of just running this block or that block. While loops allow that. They repeat the code inside a block until the answer to a condition is false. Here's an example:
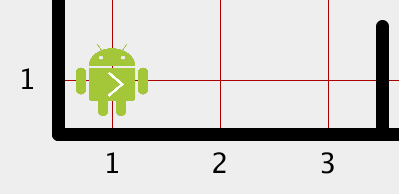
Karel is facing East at [1, 1] with 0 beepers.
while (frontIsClear()) {
println("Still Free!!!");
move();
}
println("Next to a wall");
The condition is checked once at the start of the block. Since the robot's front IS clear, the code inside the block runs all the way through once:
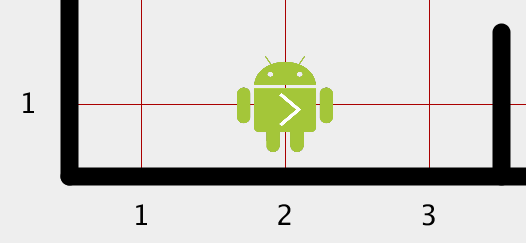
Still Free!!!
Karel is facing East at [2, 1] with 0 beepers.
Then the condition is checked again. It is still clear in front of the robot, so the code inside the block is run all the way through again:
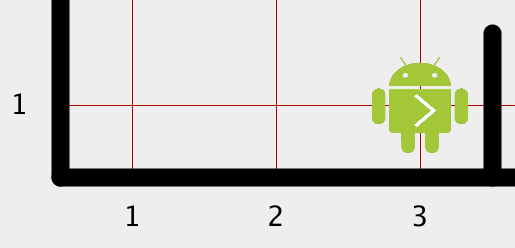
Still Free!!!
Karel is facing East at [3, 1] with 0 beepers.
Once again, the condition is checked. This time, the robot's path is blocked by a wall. The loop is now done running, and code execution continues after the block:
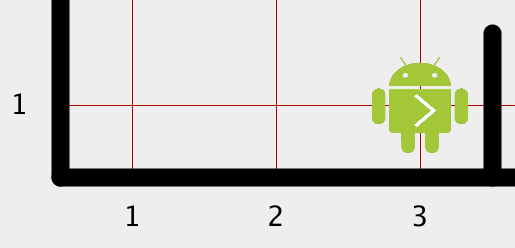
Next to a wall
While Loop FAQ
- Can while loops have
else
blocks? - No,
else
blocks are only ever attached toif
statements. - When is the condition checked?
- Once at the beginning of the loop, then once again every time the code inside the loop is done running. It is NOT checked at all times throughout the loop's execution.
- What happens if the condition is always TRUE?
- The loop will never, ever stop. This is known as an infinite loop, and it is a mistake programmers commonly make.
Recursion
It is possible to cause code to repeat by calling a method within itself, like this:
void doSomething() {
move();
doSomething();
}
This technique is called recursion.
Unfortunately, especially with new programmers, using this way of repeating code is error prone. Like in the example above, it often leads to infinite loops. It is also difficult to understand how a complicated recursive method actually works. For these reasons, avoid using recursion in your solutions.
If you want code to repeat, use a while loop, not recursion.
Conditions
To help you solve this problem, you can ask Karel yes/no questions about the state of the world. Here are your choices:
- facingEast()
- Is the robot currently pointed to the East?
- facingSouth()
- Is the robot currently pointed to the South?
- facingWest()
- Is the robot currently pointed to the West?
- facingNorth()
- Is the robot currently pointed to the North?
- frontIsClear()
- Is there a wall directly in front of the robot?
- nextToABeeper()
- Is there at least one beeper on the same intersection as the robot?
- anyBeepersInBeeperBag()
- Is the robot currently holding any beepers?