Karel Level 3 A
Description
Multiple robots have been assigned to clean up a row of beepers in front of them. Here's what the world looks like at the start:
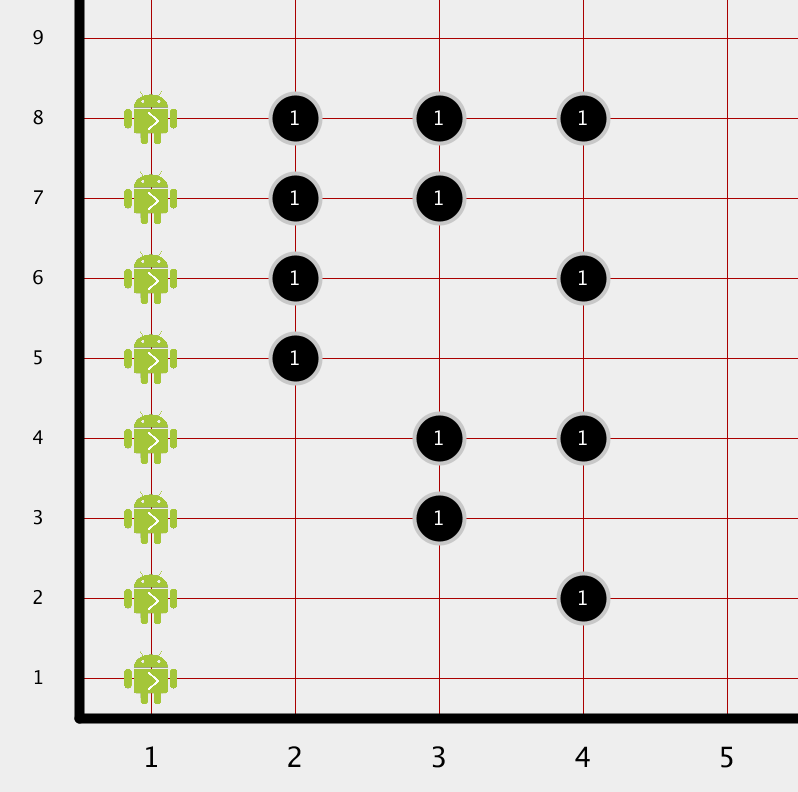
The world should look like this when all of the robots are done:
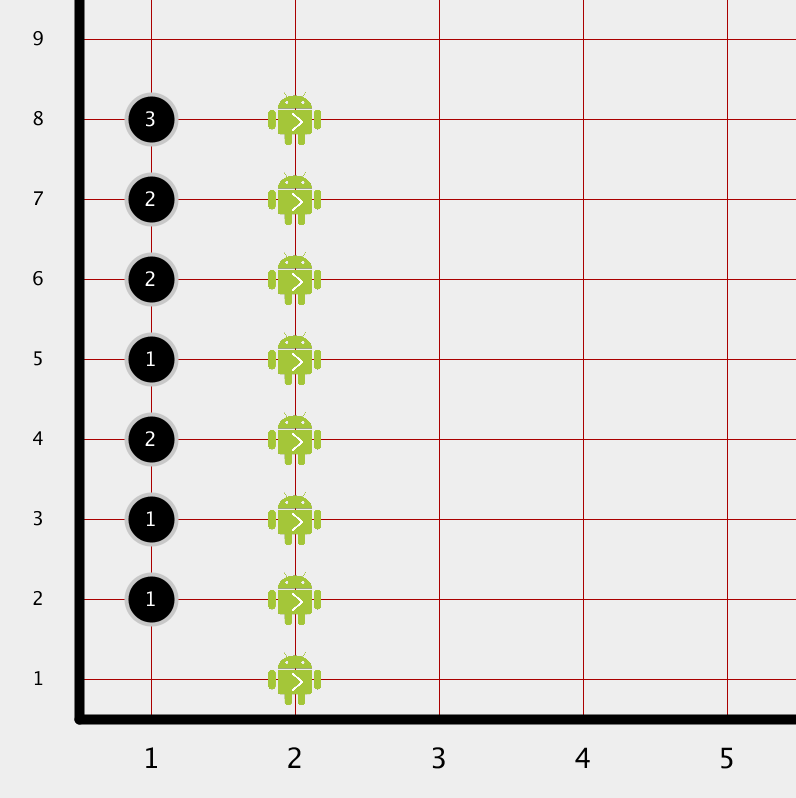
All of the robots will use the same exact code that you write! How do you make the robots do different things? Keep reading...
Conditions
To solve this problem, you can ask your robot yes/no questions about the state of the world. Here are your choices:
- facingEast()
- Is the robot currently pointed to the East?
- facingSouth()
- Is the robot currently pointed to the South?
- facingWest()
- Is the robot currently pointed to the West?
- facingNorth()
- Is the robot currently pointed to the North?
- frontIsClear()
- Is there a wall directly in front of the robot?
- nextToABeeper()
- Is there at least one beeper on the same intersection as the robot?
- anyBeepersInBeeperBag()
- Is the robot currently holding any beepers?
If Statements
If statements allow you to make part of your code conditional, meaning it only runs some of the time. Use a condition to decide whether or not the code runs. Here is an example:
if (facingEast()) {
move();
}
If the robot could understand English, the above code would translate to
"Are you facing East?
If so, move.
Otherwise, do nothing."
Else
The otherwise option is defined with an else statement. It attaches to the end of an if block like so:
if (facingNorth()) {
println("NORTH!");
} else {
println("NOT NORTH!")
}
When using both an if
and an else
, only one or the other section's code will run - never both.
Not
Sometimes it is necessary to ask the opposite of a question we already know how to ask. "Is the robot next to a wall?" is an example, it is the opposite of "Is the robot's front clear?". When the answer to the first question is YES, the answer to the second must be NO.
To avoid writing more questions, we can negate a question and ask its opposite by placing an exclamation point in front of the query, like so:
if (!frontIsClear()) {
// if I'm next to a wall
}