Karel Level 3
Description
Four robots have been tasked with duplicating a pattern of beepers. Here's what the world might start like:
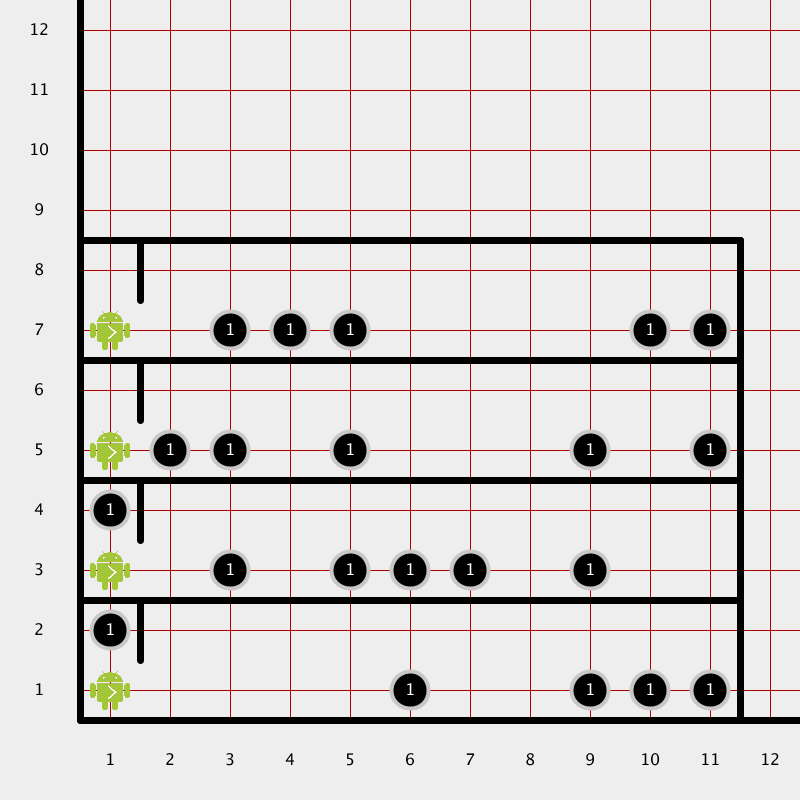
Patterns
The actual patterns will be random each time. They will always be on the same row as where the robot starts and be from column 2 to column 11.
Also, there may or may not be a beeper directly North of a robot when it starts. When there is not a beeper there the robot should create a copy of the pattern one row above it, like this:

However, when there IS a beeper directly above it at the start, it should create the OPPOSITE pattern on the row above it, like this:

The robots have been given an infinite number of beepers to start with so there is no need to pick up beepers along the way.
Solution
The final solution to the world shown earlier looks like this:
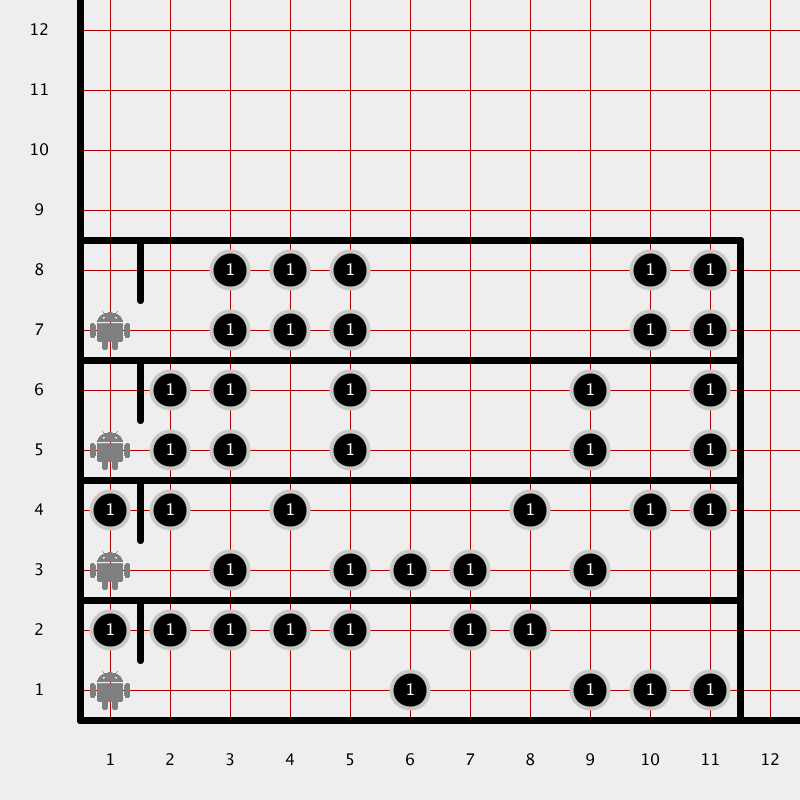
Remember to use a Top-Down approach to solve this problem!
When you think you are done, run your solution a few different times to make sure it works in all situations.
Conditions
To help you solve this problem, you can ask Karel yes/no questions about the state of the world. Here are your choices:
- facingEast()
- Is the robot currently pointed to the East?
- facingSouth()
- Is the robot currently pointed to the South?
- facingWest()
- Is the robot currently pointed to the West?
- facingNorth()
- Is the robot currently pointed to the North?
- frontIsClear()
- Is there a wall directly in front of the robot?
- nextToABeeper()
- Is there at least one beeper on the same intersection as the robot?
- anyBeepersInBeeperBag()
- Is the robot currently holding any beepers?
If Statements
If statements allow you to make part of your code conditional, meaning it only runs some of the time. Use a condition to decide whether or not the code runs. Here is an example:
if (facingEast()) {
move();
}
If the robot could understand English, the above code would translate to
"Are you facing East?
If so, move.
Otherwise, do nothing."
Else
The otherwise option is defined with an else statement. It attaches to the end of an if block like so:
if (facingNorth()) {
println("NORTH!");
} else {
println("NOT NORTH!")
}
When using both an if
and an else
, only one or the other section's code will run - never both.
Not
Sometimes it is necessary to ask the opposite of a question we already know how to ask. "Is the robot next to a wall?" is an example, it is the opposite of "Is the robot's front clear?". When the answer to the first question is YES, the answer to the second must be NO.
To avoid writing more questions, we can negate a question and ask its opposite by placing an exclamation point in front of the query, like so:
if (!frontIsClear()) {
// if I'm next to a wall
}